A Combination Of Variables Numbers And Operations
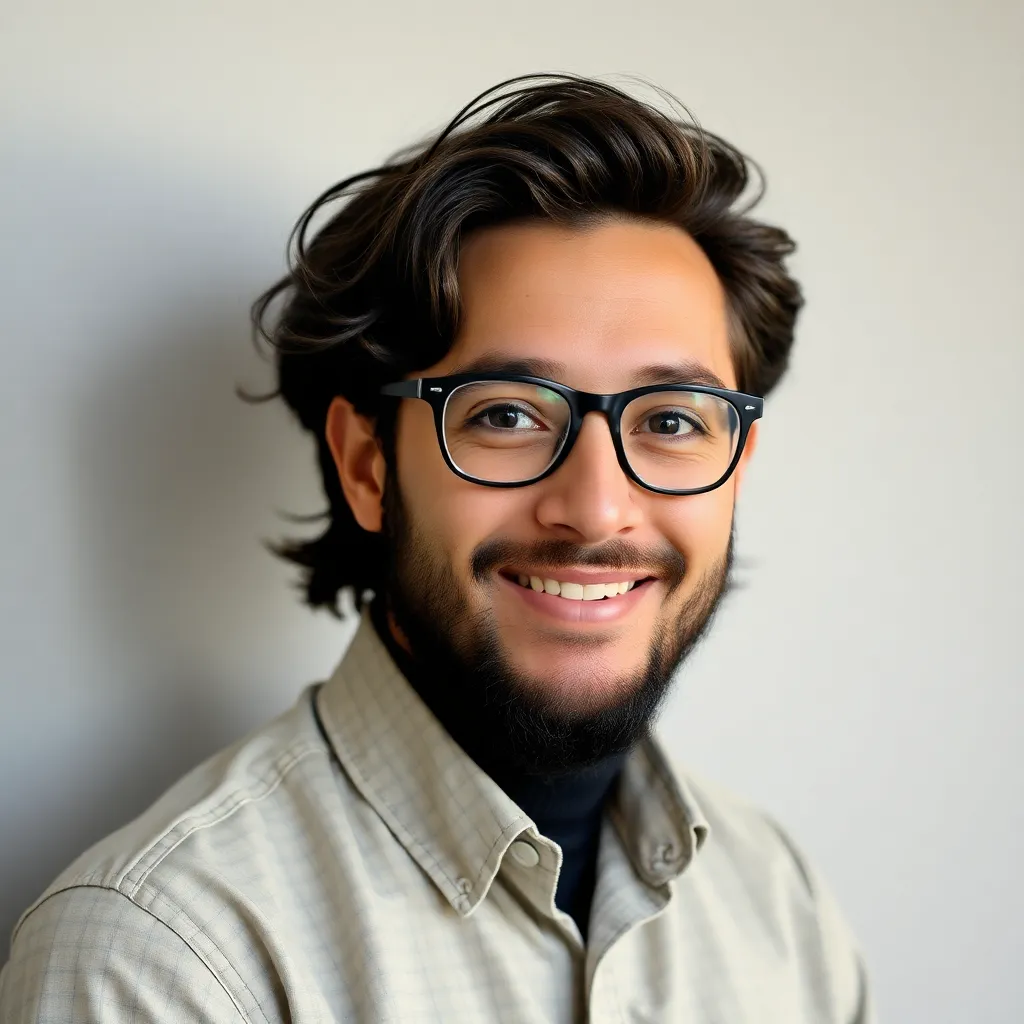
Muz Play
Apr 21, 2025 · 6 min read

Table of Contents
A Deep Dive into Combining Variables, Numbers, and Operations
The foundation of any programming language, and indeed much of mathematics, lies in the ability to combine variables, numbers, and operations to create expressions and solve problems. This seemingly simple concept unlocks a universe of computational power, allowing us to model complex systems, automate tasks, and create innovative solutions. This article will explore the intricacies of combining these elements, covering various data types, operator precedence, common pitfalls, and advanced techniques.
Understanding Variables
Variables act as containers for storing data. They're symbolic names that represent values which can change during the execution of a program. The choice of variable name should be descriptive and relevant to the data it holds (e.g., userAge
, productPrice
, totalScore
). Different programming languages have different rules for naming variables, but common practices include using lowercase letters, underscores to separate words (snake_case), or camel case (e.g., userName
).
Data Types
The type of data a variable holds determines the kind of operations that can be performed on it. Common data types include:
- Integers (int): Whole numbers without decimal points (e.g., -2, 0, 10, 1000).
- Floating-point numbers (float): Numbers with decimal points (e.g., -3.14, 0.0, 2.718).
- Strings (str): Sequences of characters (e.g., "Hello", "World", "123").
- Booleans (bool): Represent truth values, either
True
orFalse
.
Understanding data types is crucial because attempting to perform incompatible operations (e.g., adding a string to an integer without explicit type conversion) will typically result in errors.
Fundamental Operations
Arithmetic operations form the core of combining numbers and variables. These include:
- Addition (+): Adds two values together.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another. In many languages, division of integers may result in an integer truncation (the decimal part is discarded).
- Modulo (%): Returns the remainder of a division. Useful for determining even/odd numbers or cyclical patterns.
- Exponentiation (): Raises a base to a power (e.g., 2**3 = 8). The syntax may vary across languages (some use
pow(2, 3)
).
Example: Combining Numbers and Variables
Let's illustrate with a simple Python example:
price = 10.99
quantity = 5
totalCost = price * quantity
print(f"Total cost: ${totalCost:.2f}") # Output: Total cost: $54.95
This code snippet demonstrates how a variable (totalCost
) is calculated by combining a number (5) and another variable (price
) using the multiplication operator. The f-string
formatting provides a clean output.
Operator Precedence and Associativity
When multiple operations are present in an expression, the order of evaluation matters. Operator precedence determines which operations are performed first. Generally, multiplication and division have higher precedence than addition and subtraction. Parentheses ()
can be used to override the default precedence and explicitly define the order of operations.
Example:
10 + 5 * 2
evaluates to 20
(multiplication before addition).
(10 + 5) * 2
evaluates to 30
(parentheses force addition first).
Associativity dictates how operators of the same precedence are grouped. Most arithmetic operators are left-associative, meaning they are evaluated from left to right.
String Manipulation
Combining strings involves concatenation (joining strings together) and other operations specific to text processing. The plus operator (+) is commonly used for string concatenation.
Example (Python):
firstName = "John"
lastName = "Doe"
fullName = firstName + " " + lastName # Concatenation
print(f"Full Name: {fullName}") # Output: Full Name: John Doe
Many programming languages offer built-in functions and methods for more advanced string manipulation such as substring extraction, searching, replacing, and formatting.
Type Conversion (Casting)
It's often necessary to convert data from one type to another. This process is called type casting or type conversion. For example, you might need to convert a string representation of a number into an integer or a floating-point number before performing arithmetic operations.
Example (Python):
stringNumber = "123"
integerNumber = int(stringNumber) # Type conversion to integer
floatNumber = float(stringNumber) # Type conversion to float
sumOfNumbers = integerNumber + floatNumber #Now possible
print(f"The sum is {sumOfNumbers}")
Improper type conversion can lead to errors, so it's essential to understand the rules and limitations of type conversion in your chosen programming language.
Advanced Techniques
Beyond the basics, several advanced techniques involve combining variables, numbers, and operations:
1. Conditional Expressions (If-Else Statements)
Conditional expressions allow you to execute different blocks of code based on certain conditions. They use comparison operators (e.g., ==, !=, <, >, <=, >=) to evaluate truth values.
Example (Python):
age = 20
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
2. Loops (For and While Loops)
Loops are used to repeat a block of code multiple times. For
loops iterate over a sequence (e.g., a list, array, or string), while while
loops continue as long as a condition is true.
Example (Python):
for i in range(5): #For loop iterating 5 times
print(i)
count = 0
while count < 5:
print(count)
count += 1
3. Functions
Functions encapsulate a block of code that performs a specific task. They improve code organization, reusability, and readability. Functions can accept input parameters (arguments) and return values.
Example (Python):
def calculateArea(length, width):
return length * width
area = calculateArea(5, 10)
print(f"The area is: {area}")
4. Arrays and Lists
Arrays and lists (similar data structures in different languages) allow you to store collections of data elements. Operations on arrays/lists often involve iterating through the elements and performing calculations or manipulations.
Example (Python):
numbers = [1, 2, 3, 4, 5]
sumOfNumbers = sum(numbers) #Built in sum function
print(f"The sum of the numbers is: {sumOfNumbers}")
5. Working with Libraries and Modules
Programming languages provide extensive libraries and modules that offer pre-built functions and classes for various tasks, including mathematical operations, string manipulation, data analysis, and more. Leveraging these resources significantly simplifies development and enhances efficiency.
Common Pitfalls and Debugging
When combining variables, numbers, and operations, several common pitfalls can lead to errors:
- Type errors: Attempting to perform incompatible operations between different data types.
- Operator precedence errors: Incorrectly interpreting the order of operations, leading to unexpected results.
- Off-by-one errors: Incorrectly handling loop boundaries or array indices.
- Logic errors: Flaws in the program's logic that produce incorrect output.
Debugging involves systematically identifying and fixing these errors. Techniques include using print statements to inspect variable values, using a debugger to step through code execution, and carefully reviewing the code's logic.
Conclusion
The ability to combine variables, numbers, and operations is fundamental to programming. Mastering this skill unlocks the potential to create sophisticated programs that solve complex problems and automate tasks efficiently. Understanding data types, operator precedence, type conversion, and advanced techniques is crucial for building robust and reliable software. Careful attention to detail and effective debugging strategies are vital for avoiding common pitfalls and ensuring the correctness of your code. Continuous learning and practice are key to advancing your proficiency in this essential aspect of programming.
Latest Posts
Latest Posts
-
Determine The Products Of This First Reaction
Apr 21, 2025
-
Groups Of Cells Sharing Similar Morphology And Function Form Tissue
Apr 21, 2025
-
Can A Rate Constant Be Negative
Apr 21, 2025
-
Can A Moving Object Be In Equilibrium
Apr 21, 2025
-
The Posterior Rami Of The Spinal Nerves Serve The
Apr 21, 2025
Related Post
Thank you for visiting our website which covers about A Combination Of Variables Numbers And Operations . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.