Find The Class With The Least Number Of Data Values
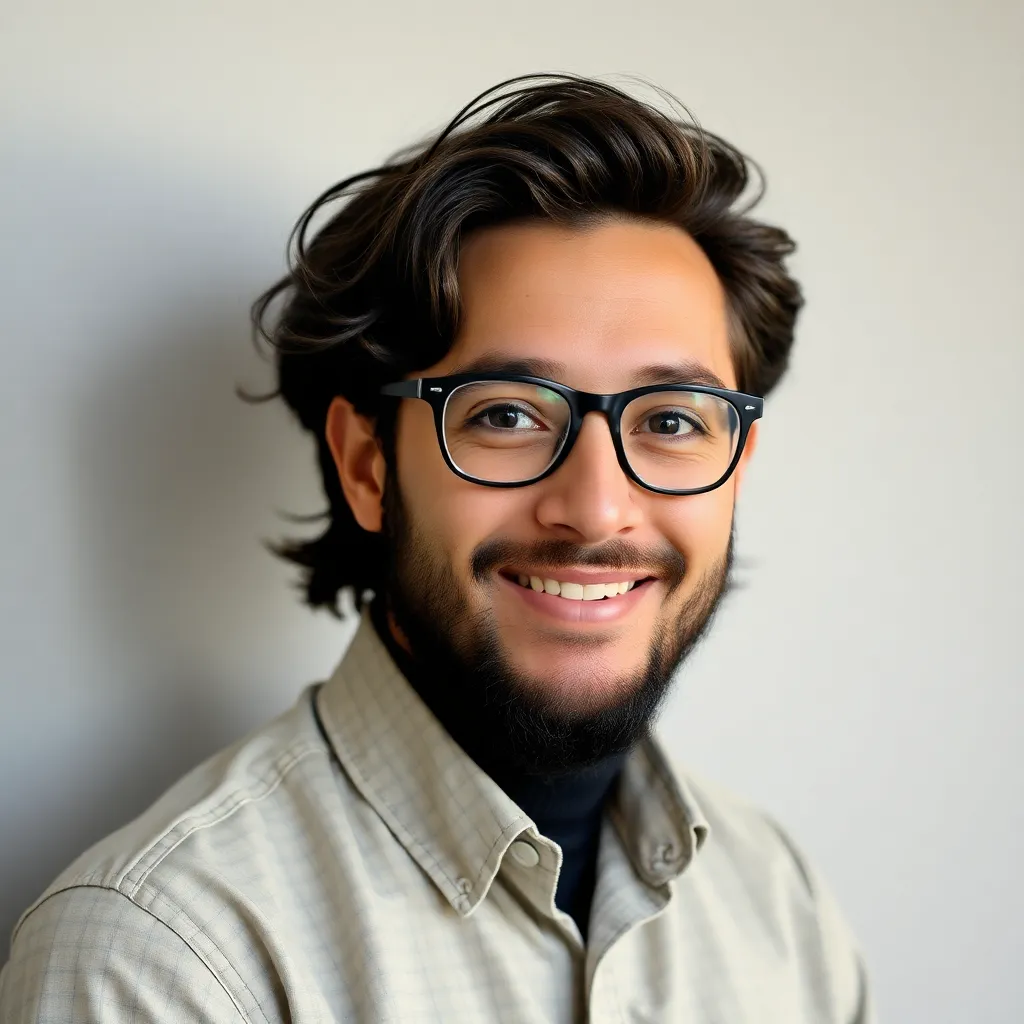
Muz Play
May 10, 2025 · 6 min read
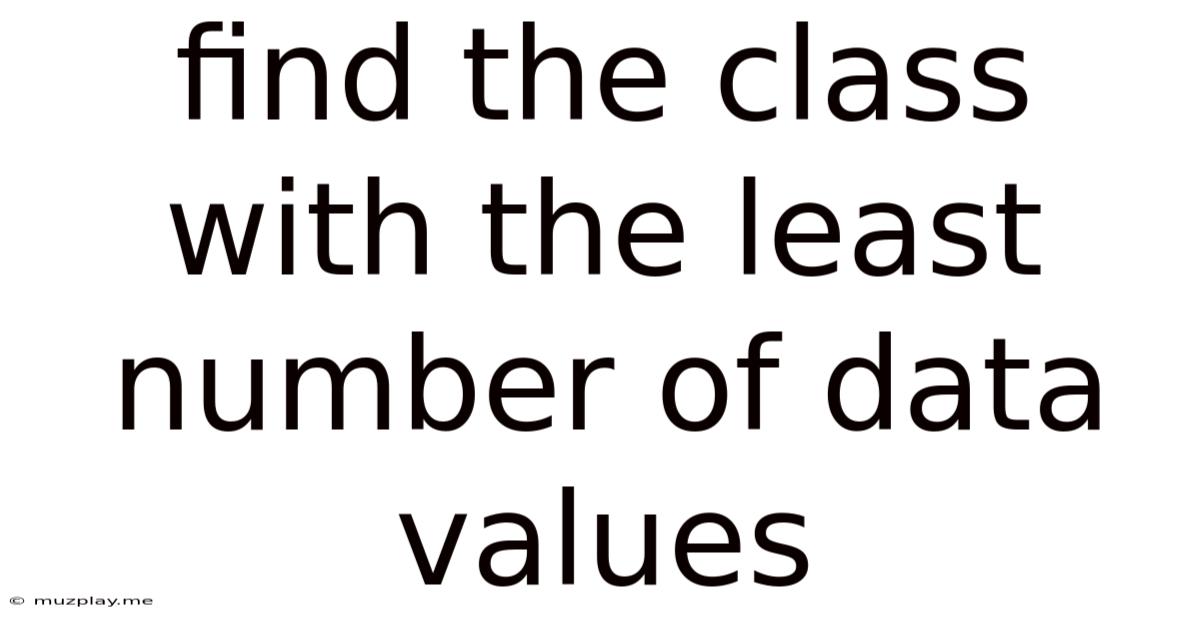
Table of Contents
Finding the Class with the Least Number of Data Values: A Comprehensive Guide
Finding the class with the fewest data points within a dataset is a common task in data analysis and statistics. This seemingly simple problem can become complex depending on the dataset's size, structure, and the methods used for data organization and classification. This comprehensive guide will explore various approaches to identifying the class with the least number of data values, catering to different levels of data analysis expertise. We'll cover fundamental concepts, practical examples using Python, and strategies for handling diverse data scenarios.
Understanding Data Classification and Frequency Distribution
Before diving into the methods, it's crucial to understand the concepts of data classification and frequency distribution. Data classification involves grouping similar data points into distinct categories or classes. A frequency distribution then summarizes how many data points fall into each class. This is often represented visually using histograms or frequency tables. Identifying the class with the least number of data values essentially means finding the class with the lowest frequency in the frequency distribution.
Types of Data and Classification Methods
The approach to finding the class with the least number of data values depends heavily on the type of data you're dealing with:
-
Categorical Data: This type of data represents categories or groups, such as colors (red, blue, green), types of animals (cat, dog, bird), or customer segments (high-value, medium-value, low-value). Finding the least frequent class is straightforward here; simply count the occurrences of each category.
-
Numerical Data: Numerical data represents quantities, such as age, height, weight, or income. To find the class with the least number of data values, you first need to classify the numerical data into intervals or bins. Histograms are commonly used to visualize this classification. The choice of bin width significantly impacts the results. A narrower bin width provides more detail but might lead to many classes with very few data points. A wider bin width might obscure important details.
-
Mixed Data: Datasets can contain both categorical and numerical data. In such cases, you might need to perform separate analyses for different data types or create composite classes that consider both categorical and numerical features.
Methods for Finding the Least Frequent Class
Several methods can be employed to identify the class with the fewest data values. The best approach depends on the size and structure of your dataset and the tools you're comfortable using.
1. Manual Counting (for small datasets)
For very small datasets, manual counting is feasible. Simply create a frequency table by visually inspecting the data and counting the occurrences of each class. The class with the smallest count represents the class with the least number of data values. This method is impractical for large datasets.
2. Using Python Dictionaries (for moderate datasets)
Python dictionaries provide an efficient way to count the frequency of classes. A dictionary is used where keys represent the classes, and the values represent the count of data points in each class.
data = ['A', 'B', 'A', 'C', 'B', 'A', 'D', 'B', 'A']
class_counts = {}
for item in data:
if item in class_counts:
class_counts[item] += 1
else:
class_counts[item] = 1
min_class = min(class_counts, key=class_counts.get)
min_count = class_counts[min_class]
print(f"The class with the least number of data values is: {min_class} ({min_count})")
This code snippet iterates through the data, counting occurrences of each class and storing them in a dictionary. The min()
function, along with the key
argument, efficiently finds the key (class) with the minimum value (count).
3. Using Pandas (for large datasets)
For large datasets, the Pandas library in Python offers a powerful and efficient solution. Pandas value_counts()
method directly calculates the frequency of each unique value in a Series or column.
import pandas as pd
data = pd.Series(['A', 'B', 'A', 'C', 'B', 'A', 'D', 'B', 'A'])
class_counts = data.value_counts()
min_class = class_counts.idxmin()
min_count = class_counts.min()
print(f"The class with the least number of data values is: {min_class} ({min_count})")
This code uses Pandas to efficiently compute the class frequencies. idxmin()
returns the index (class) with the minimum value, and .min()
returns the minimum value itself. Pandas handles large datasets significantly faster than manual counting or using dictionaries alone.
4. Handling Numerical Data with Histograms and Binning
For numerical data, you'll need to create bins (intervals) to group the data. The numpy.histogram()
function in Python is useful for this purpose.
import numpy as np
data = np.array([1, 2, 3, 1, 2, 4, 5, 1, 2, 2, 6, 1])
hist, bin_edges = np.histogram(data, bins=range(min(data), max(data) + 2))
min_index = np.argmin(hist)
min_bin = (bin_edges[min_index], bin_edges[min_index+1])
min_count = hist[min_index]
print(f"The bin with the least number of data values is: {min_bin} ({min_count})")
This code creates a histogram, identifying the bin with the lowest frequency. Note that the binning process (choosing the number of bins) can significantly influence the results. Experiment with different binning strategies to find the most meaningful representation of your data.
Advanced Considerations and Challenges
The process of finding the least frequent class becomes more complex in certain scenarios:
-
Handling Ties: What if multiple classes have the same minimum frequency? The code examples above will typically return only one of the tied classes. You might need to modify the code to return all classes with the minimum frequency if this situation is relevant to your analysis.
-
Missing Data: Missing values in your dataset can skew the frequency counts. Carefully handle missing data (e.g., imputation or removal) before calculating frequencies to avoid inaccurate results.
-
High-Dimensional Data: For datasets with many features, dimensionality reduction techniques may be needed before applying the methods described above.
Conclusion
Finding the class with the least number of data values is a crucial step in various data analysis tasks. Choosing the appropriate method depends on the dataset's characteristics and the level of detail required. For small datasets, manual counting suffices. For moderate to large datasets, using Python dictionaries or the powerful Pandas library is significantly more efficient. Remember to consider data types, handling ties and missing values, and the impact of binning when dealing with numerical data. By understanding these concepts and techniques, you'll be equipped to effectively analyze your data and draw meaningful insights. Remember that data visualization techniques, such as histograms and bar charts, can greatly enhance the interpretation of your findings, offering a clear visual representation of the class frequencies and helping to identify outliers or unexpected patterns. This holistic approach will improve both the accuracy and the understanding of your data analysis.
Latest Posts
Latest Posts
-
Is Salt On The Periodic Table
May 10, 2025
-
What Is The General Chemical Equation For An Endothermic Reaction
May 10, 2025
-
Round Each Number To Three Significant Figures
May 10, 2025
-
Why Are Chromosomes Important For Mitosis
May 10, 2025
-
Energy Diagram For Sp3 Hybridized Oxygen
May 10, 2025
Related Post
Thank you for visiting our website which covers about Find The Class With The Least Number Of Data Values . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.