How Do You Find The Length Of A Vector
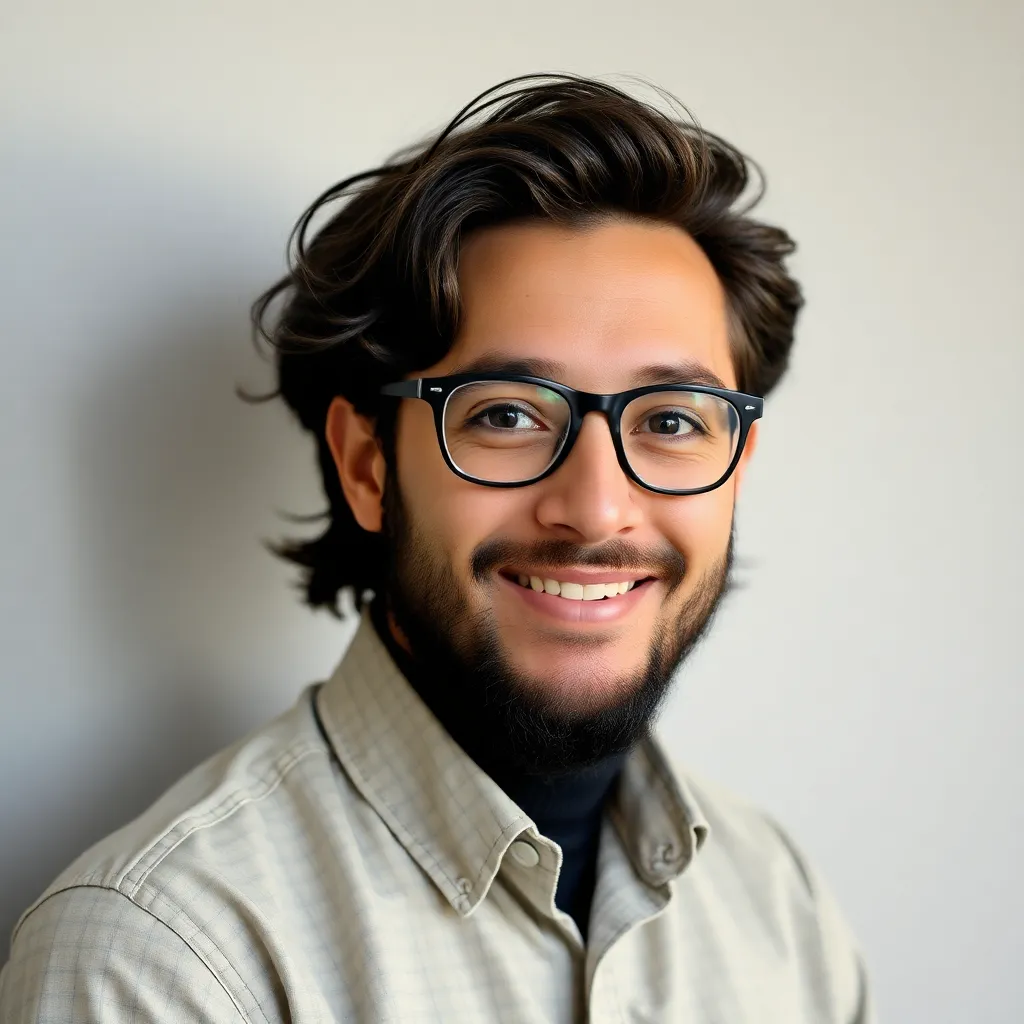
Muz Play
Mar 23, 2025 · 6 min read
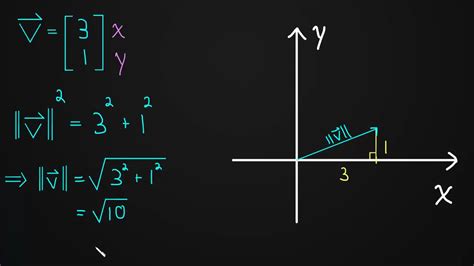
Table of Contents
How Do You Find the Length of a Vector? A Comprehensive Guide
Finding the length of a vector, also known as its magnitude or norm, is a fundamental operation in linear algebra and has wide-ranging applications in physics, computer graphics, machine learning, and many other fields. This comprehensive guide will delve into various methods for calculating vector length, catering to different levels of understanding, from introductory concepts to more advanced techniques.
Understanding Vectors
Before diving into the methods for calculating vector length, let's establish a clear understanding of what a vector is. A vector is a mathematical object that possesses both magnitude (length) and direction. It's often represented visually as an arrow, where the arrow's length represents the magnitude, and the arrow's orientation indicates the direction. Vectors can be represented in various ways, most commonly as:
- Geometrically: As arrows in space.
- Algebraically: As ordered lists of numbers (components), such as (x, y) in 2D space or (x, y, z) in 3D space.
Calculating Vector Length: The Basic Approach
The most common method for calculating the length of a vector involves the Pythagorean theorem, extended to higher dimensions. Let's break it down:
Two-Dimensional Vectors
For a 2D vector v = (x, y), the length (magnitude) ||v|| is given by:
||v|| = √(x² + y²)
This formula directly applies the Pythagorean theorem to the right-angled triangle formed by the vector's components. The x and y components are the legs of the triangle, and the vector's length is the hypotenuse.
Example:
Let's say we have a vector v = (3, 4). The length is:
||v|| = √(3² + 4²) = √(9 + 16) = √25 = 5
Three-Dimensional Vectors
The concept extends seamlessly to three dimensions. For a 3D vector v = (x, y, z), the length is:
||v|| = √(x² + y² + z²)
This is a direct extension of the Pythagorean theorem to three dimensions.
Example:
For a vector v = (1, 2, 2), the length is:
||v|| = √(1² + 2² + 2²) = √(1 + 4 + 4) = √9 = 3
N-Dimensional Vectors
The principle generalizes to vectors of any dimension (n). For an n-dimensional vector v = (x₁, x₂, ..., xₙ), the length is:
||v|| = √(x₁² + x₂² + ... + xₙ²)
This is also known as the Euclidean norm or L2 norm. It represents the straight-line distance from the origin to the point represented by the vector.
Beyond the Euclidean Norm: Other Types of Vector Norms
While the Euclidean norm is the most commonly used, other norms exist, each defining length in a slightly different way. These are particularly useful in specific mathematical and computational contexts.
L1 Norm (Manhattan Distance)
The L1 norm, also known as the Manhattan distance or taxicab geometry, sums the absolute values of the vector components:
||v||₁ = |x₁| + |x₂| + ... + |xₙ|
Imagine navigating a city grid; you can only move along streets, not diagonally. The L1 norm represents the total distance traveled.
L∞ Norm (Maximum Norm)
The L∞ norm is the maximum absolute value among the vector components:
||v||∞ = max(|x₁|, |x₂|, ..., |xₙ|)
This norm is useful when you are interested in the component with the largest magnitude.
Lp Norm (Generalized Norm)
The Euclidean norm (L2) and the L1 norm are specific cases of the more general Lp norm:
||v||ₚ = (|x₁|ᵖ + |x₂|ᵖ + ... + |xₙ|ᵖ)^(1/p)
where p is a positive real number. The Euclidean norm is obtained when p=2, and the L1 norm when p=1. The L∞ norm is obtained as the limit as p approaches infinity.
Applications of Vector Length Calculation
The ability to determine the length of a vector is crucial in a wide array of applications. Here are a few examples:
Physics
- Velocity and Speed: A velocity vector describes both the speed and direction of an object. The magnitude of the velocity vector represents the speed.
- Force: Forces are vector quantities. The magnitude of a force vector represents the strength of the force.
- Displacement: The magnitude of a displacement vector represents the distance between two points.
Computer Graphics
- Normalization: Often, vectors need to be normalized—scaled to have a length of 1. This is done by dividing each component of the vector by its magnitude. Normalized vectors are essential for representing directions without considering magnitude.
- Distance Calculations: Determining the distance between points in 3D space is a fundamental operation in computer graphics, and it relies directly on vector length calculations.
- Lighting and Shading: Lighting calculations in computer graphics frequently involve vector operations, including determining the lengths of vectors to calculate light intensity.
Machine Learning
- Feature Scaling: Many machine learning algorithms benefit from feature scaling, where the magnitude of features is normalized to prevent features with larger values from dominating the learning process.
- Cosine Similarity: The cosine similarity between two vectors measures the angle between them, independent of their magnitudes. Calculating vector lengths is a crucial step in computing cosine similarity.
- Distance Metrics: Various distance metrics used in clustering and classification algorithms (e.g., k-nearest neighbors) rely on calculating the distances between data points represented as vectors.
Other Applications
- Robotics: Calculating the distance between the robot arm and an object is essential for precise movements.
- Signal Processing: Vector norms are employed in analyzing signals and filtering noise.
- Quantum Mechanics: Vectors represent quantum states, and the length of a state vector is related to the probability of finding the system in that particular state.
Programming Vector Length Calculations
Calculating vector lengths is straightforward in many programming languages. Here's a simple example in Python:
import math
def vector_length(vector):
"""Calculates the Euclidean length of a vector."""
return math.sqrt(sum(x**2 for x in vector))
# Example usage:
v = [3, 4]
length = vector_length(v)
print(f"The length of vector {v} is: {length}")
v = [1, 2, 2]
length = vector_length(v)
print(f"The length of vector {v} is: {length}")
This Python function utilizes a generator expression for efficient summation of squared components, making it suitable for high-dimensional vectors. Similar functions can be easily implemented in other languages like C++, Java, or JavaScript, leveraging built-in mathematical functions.
Conclusion
Finding the length of a vector is a fundamental concept with widespread applications across diverse fields. Understanding the different methods for calculating vector length, including the Euclidean norm and other types of norms, equips you with a powerful tool for tackling various mathematical and computational problems. From basic physics to advanced machine learning algorithms, the ability to determine vector magnitude is essential for solving real-world problems effectively. The examples and code snippets provided demonstrate the practical implementation of these concepts, making it easier to integrate vector length calculations into your own projects. Remember to choose the appropriate norm based on the specific application and context of your problem.
Latest Posts
Latest Posts
-
Is Boil A Physical Or Chemical Change
Mar 25, 2025
-
How To Write All Real Numbers In Interval Notation
Mar 25, 2025
-
What Are Rows Called On The Periodic Table
Mar 25, 2025
-
What Group Defines Themselves Through A Rejection Of The Mainstream
Mar 25, 2025
-
Transfer Function Of An Rc Circuit
Mar 25, 2025
Related Post
Thank you for visiting our website which covers about How Do You Find The Length Of A Vector . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.