How To Find Eigenvectors Of A 4x4 Matrix
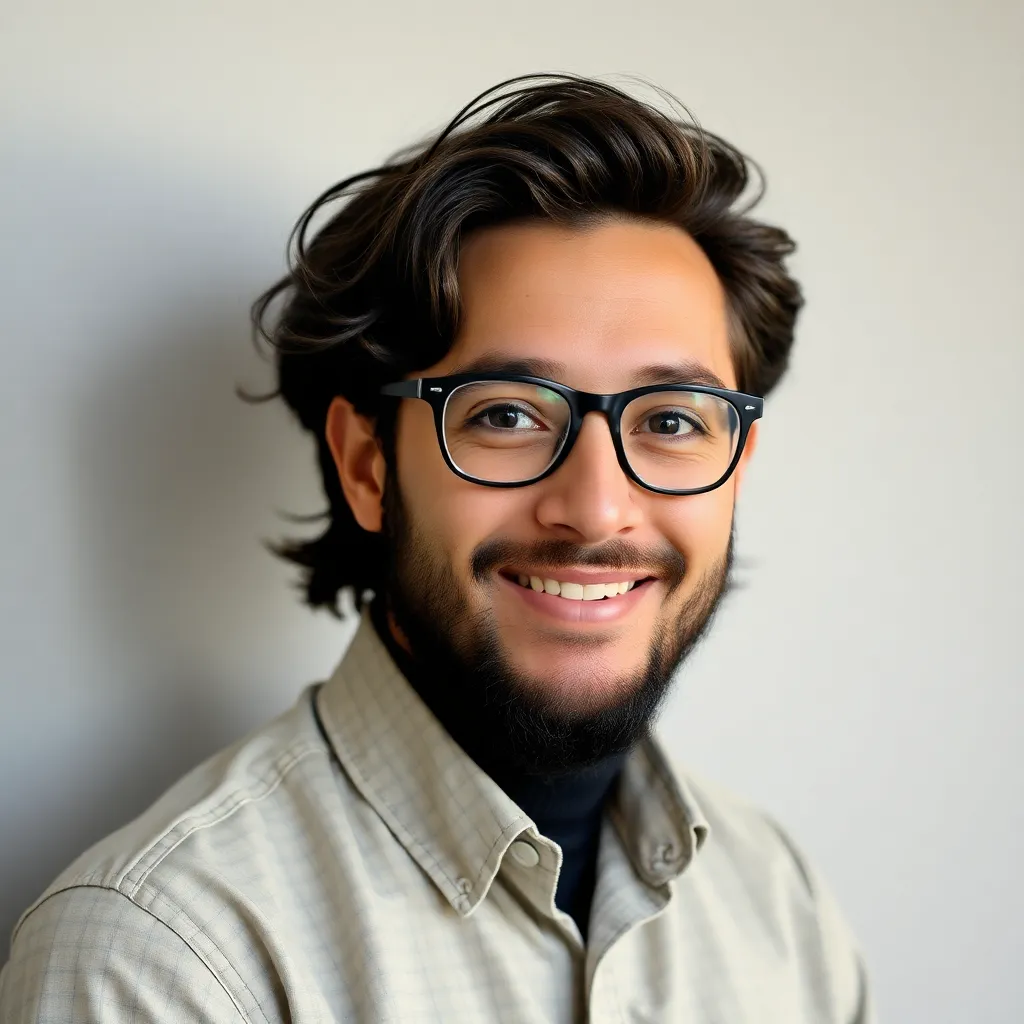
Muz Play
Apr 26, 2025 · 6 min read

Table of Contents
How to Find Eigenvectors of a 4x4 Matrix: A Comprehensive Guide
Finding eigenvectors of a 4x4 matrix might seem daunting, but with a systematic approach and a solid understanding of the underlying concepts, it becomes manageable. This comprehensive guide breaks down the process step-by-step, covering both the theoretical foundations and practical computational techniques. We'll explore various methods, emphasizing clarity and practicality.
Understanding Eigenvalues and Eigenvectors
Before diving into the calculations, let's solidify our understanding of the core concepts. Eigenvectors are special vectors that, when multiplied by a matrix, only change in scale (magnitude), not direction. The scaling factor is the eigenvalue. Mathematically, this relationship is expressed as:
Av = λv
where:
- A is the 4x4 matrix
- v is the eigenvector
- λ is the eigenvalue
This equation essentially states that applying the transformation represented by matrix A to the eigenvector v results in a vector that's parallel to v (same direction). The eigenvalue λ quantifies the scaling effect.
Step-by-Step Process: Finding Eigenvectors of a 4x4 Matrix
The process generally involves these key steps:
1. Finding the Eigenvalues
This is the most challenging part. To find the eigenvalues, we need to solve the characteristic equation:
det(A - λI) = 0
where:
- det() denotes the determinant
- I is the 4x4 identity matrix
This equation results in a fourth-degree polynomial equation in λ. Solving this polynomial can be complex and may require numerical methods for larger matrices. Let's consider a hypothetical 4x4 matrix:
A = [[2, 1, 0, 0],
[1, 2, 0, 0],
[0, 0, 3, 1],
[0, 0, 1, 3]]
Calculating the determinant (A - λI) and setting it to zero would give us a fourth-degree polynomial. Finding the roots (λ values) of this polynomial will be our eigenvalues. In this specific example, because of the block diagonal structure, the characteristic equation conveniently factors into two quadratic equations, simplifying the process. However, this isn't always the case.
Methods for Solving the Characteristic Equation:
- Analytical Methods: For simple matrices, you might be able to factor the polynomial directly. However, this becomes increasingly difficult for higher-order polynomials.
- Numerical Methods: For larger or more complex matrices, numerical methods are essential. These methods approximate the roots (eigenvalues) using iterative algorithms. Common methods include the Newton-Raphson method, the secant method, and various eigenvalue algorithms designed specifically for matrices (like the QR algorithm). Software packages like MATLAB, Python's NumPy/SciPy, and others offer built-in functions to efficiently compute eigenvalues.
Let's assume, for the sake of this example, that we've found the eigenvalues to be λ₁ = 1, λ₂ = 3, λ₃ = 2, λ₄ = 4.
2. Finding the Eigenvectors for Each Eigenvalue
Once we have the eigenvalues, we can find the corresponding eigenvectors. For each eigenvalue λᵢ, we solve the following equation:
(A - λᵢI)vᵢ = 0
This is a system of four linear homogeneous equations. The solution to this system will be the eigenvector vᵢ corresponding to eigenvalue λᵢ.
Solving the System of Equations:
This typically involves techniques like Gaussian elimination or row reduction to find the null space (kernel) of the matrix (A - λᵢI). The null space represents all vectors that, when multiplied by (A - λᵢI), result in the zero vector. This null space will contain the eigenvector(s) for that eigenvalue.
Let's illustrate with λ₁ = 1:
(A - I)v₁ = 0
Substituting the matrix A, we have:
[[1, 1, 0, 0],
[1, 1, 0, 0],
[0, 0, 2, 1],
[0, 0, 1, 2]] v₁ = 0
Solving this system using row reduction would yield a solution for v₁ (a non-trivial solution, as the trivial solution v₁=0 is always present but uninteresting). The solution will typically have one or more free variables, leading to a family of eigenvectors, all scalar multiples of each other. We can choose a convenient normalization to represent the eigenvector.
Repeating for Other Eigenvalues:
We repeat this process for each eigenvalue (λ₂, λ₃, λ₄) to obtain the corresponding eigenvectors (v₂, v₃, v₄). Remember, the number of linearly independent eigenvectors associated with each eigenvalue depends on the algebraic and geometric multiplicity of that eigenvalue. If the algebraic multiplicity is greater than one, you might find multiple linearly independent eigenvectors for that eigenvalue, or you might find only one. This nuance relates to the concepts of diagonalizability and defective matrices.
3. Verification
Once you've found the eigenvalues and eigenvectors, it's crucial to verify your results. Substitute each eigenvalue and its corresponding eigenvector back into the original equation:
Av = λv
If the equation holds true (within a reasonable margin of error for numerical approximations), your calculations are correct.
Advanced Considerations and Special Cases
- Degeneracy (Repeated Eigenvalues): When eigenvalues are repeated, finding linearly independent eigenvectors can be more challenging. The number of linearly independent eigenvectors associated with a repeated eigenvalue is equal to its geometric multiplicity. If this is less than its algebraic multiplicity, the matrix is defective, and it is not diagonalizable.
- Complex Eigenvalues: For some matrices, especially those representing rotations or oscillations, you may encounter complex eigenvalues and eigenvectors. The methods remain similar, but you'll be dealing with complex numbers.
- Numerical Stability: Numerical methods for solving polynomial equations and systems of linear equations can be susceptible to numerical errors, especially for ill-conditioned matrices. Choosing appropriate algorithms and considering error analysis is important.
- Software Tools: Utilize mathematical software packages (MATLAB, Python with SciPy/NumPy, etc.) to handle the computational burden, especially for larger matrices. These tools provide optimized algorithms and functions for eigenvalue and eigenvector calculations.
Example using Python (NumPy and SciPy)
Python, with its powerful libraries NumPy and SciPy, simplifies the process considerably. Here's a code snippet demonstrating the calculation:
import numpy as np
from scipy.linalg import eig
# Define the 4x4 matrix
A = np.array([[2, 1, 0, 0],
[1, 2, 0, 0],
[0, 0, 3, 1],
[0, 0, 1, 3]])
# Calculate eigenvalues and eigenvectors
eigenvalues, eigenvectors = eig(A)
# Print the results
print("Eigenvalues:", eigenvalues)
print("Eigenvectors:\n", eigenvectors)
This code utilizes the eig()
function from SciPy's linalg
module, which efficiently computes eigenvalues and eigenvectors. The output will provide the eigenvalues as a NumPy array and the eigenvectors as a matrix where each column represents an eigenvector.
Conclusion
Finding eigenvectors of a 4x4 matrix, while computationally intensive, becomes manageable with a structured approach. Understanding the underlying theory, employing appropriate numerical methods when necessary, and leveraging software tools are crucial for efficient and accurate computation. Remember to always verify your results to ensure accuracy. The process, although detailed, is fundamentally about solving a system of linear equations and understanding the underlying mathematical principles. This guide has equipped you with the knowledge and tools to tackle this important linear algebra problem effectively.
Latest Posts
Latest Posts
-
What Type Of Ions Do Transition Metals Form
Apr 26, 2025
-
Do Both Prokaryotes And Eukaryotes Have Ribosomes
Apr 26, 2025
-
Difference Between Starch And Cellulose And Glycogen
Apr 26, 2025
-
De Donde Proviene La Religion Yoruba
Apr 26, 2025
-
Why Is Density Important To Life
Apr 26, 2025
Related Post
Thank you for visiting our website which covers about How To Find Eigenvectors Of A 4x4 Matrix . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.