When Is A Conditional Statement False
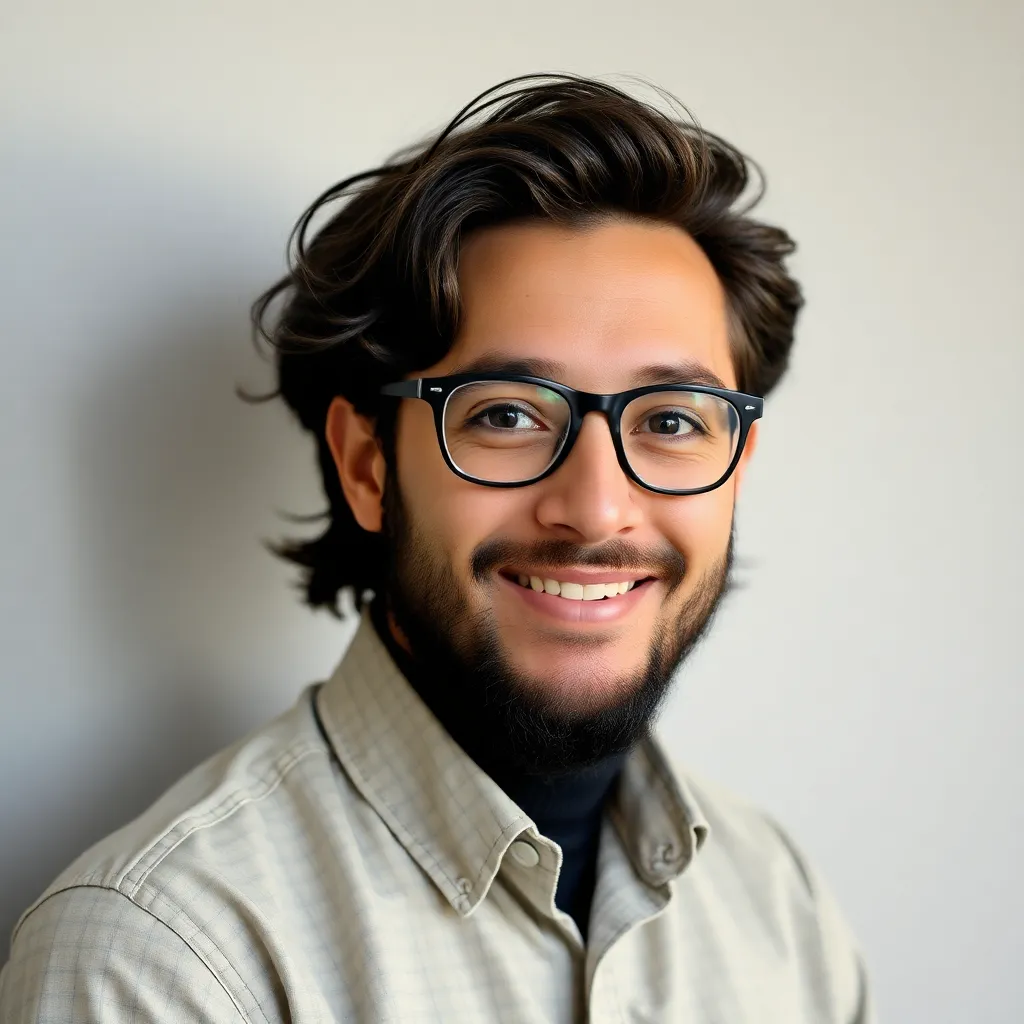
Muz Play
May 12, 2025 · 6 min read
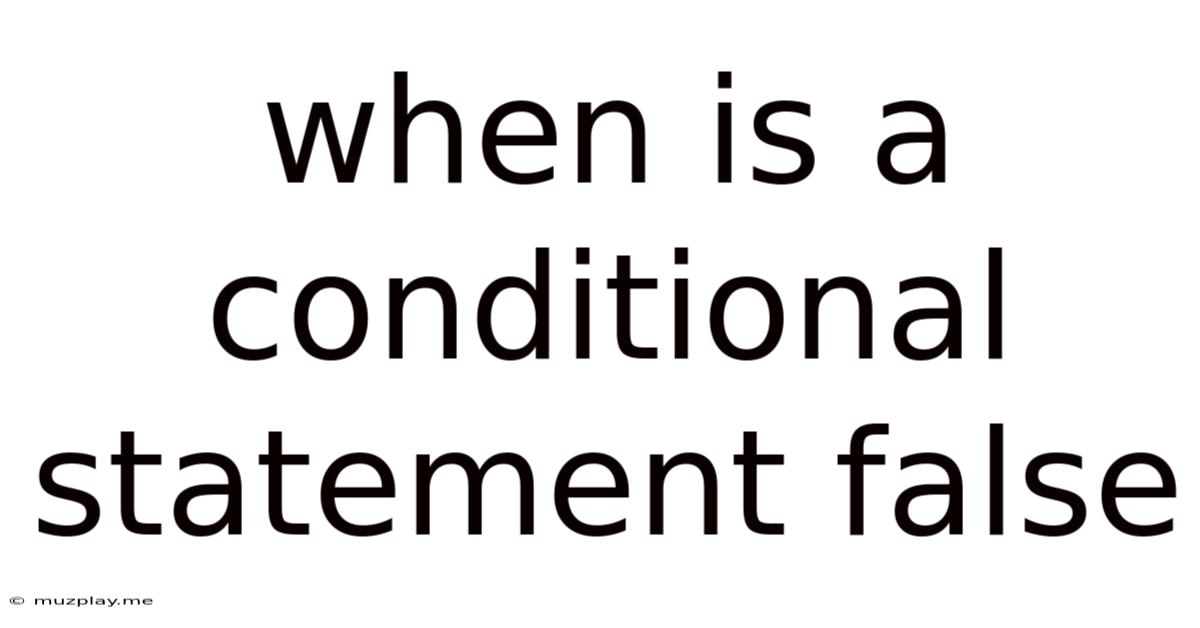
Table of Contents
When is a Conditional Statement False? A Deep Dive into Boolean Logic
Conditional statements, the backbone of programming logic, dictate the flow of execution based on whether a condition is true or false. Understanding when a conditional statement evaluates to false is crucial for writing robust and error-free code. This comprehensive guide delves into the nuances of boolean logic and explores various scenarios where conditional statements return a false value. We'll cover different programming languages, common pitfalls, and best practices to ensure your code functions as intended.
Understanding Boolean Logic: The Foundation of Conditional Statements
At the heart of every conditional statement lies boolean logic. Boolean logic operates on two values: true and false. These values represent the outcome of a condition, determining whether a block of code will execute. Conditional statements use comparison operators and logical operators to evaluate conditions and produce a boolean result.
Comparison Operators: Evaluating Expressions
Comparison operators compare two values and return a boolean value. Common comparison operators include:
==
(Equal to): Checks if two values are equal. Note the difference between==
(comparison) and=
(assignment).!=
(Not equal to): Checks if two values are not equal.>
(Greater than): Checks if the left operand is greater than the right operand.<
(Less than): Checks if the left operand is less than the right operand.>=
(Greater than or equal to): Checks if the left operand is greater than or equal to the right operand.<=
(Less than or equal to): Checks if the left operand is less than or equal to the right operand.
Example (Python):
x = 10
y = 5
print(x == y) # Output: False
print(x != y) # Output: True
print(x > y) # Output: True
print(x < y) # Output: False
Logical Operators: Combining Conditions
Logical operators combine multiple boolean expressions to create more complex conditions. Common logical operators include:
&&
orand
(Logical AND): Returns true only if both operands are true.||
oror
(Logical OR): Returns true if at least one operand is true.!
ornot
(Logical NOT): Inverts the boolean value of its operand (true becomes false, and vice-versa).
Example (JavaScript):
let a = true;
let b = false;
console.log(a && b); // Output: false
console.log(a || b); // Output: true
console.log(!a); // Output: false
Scenarios Where Conditional Statements Evaluate to False
A conditional statement evaluates to false when the condition it evaluates is logically false. Let's explore common scenarios:
1. Incorrect Comparison Operators
Using the wrong comparison operator is a frequent cause of false conditionals. For instance, accidentally using =
instead of ==
in a comparison will always assign a value and return true (unless assigning false
).
Example (C++):
int age = 25;
if (age = 30) { // Assignment, not comparison! This will always be true because it assigns 30 to age.
// This code will always execute.
}
if (age == 30) { // Correct comparison. This will be false.
// This code will not execute.
}
2. Type Mismatches
Comparing values of different data types can lead to unexpected results. Some languages perform implicit type conversion, while others might throw errors. It's crucial to ensure data types are consistent before comparison.
Example (Java):
String str = "10";
int num = 10;
if (str == num) { // This will be false because a String and an integer are compared directly.
// This code will not execute.
}
if (Integer.parseInt(str) == num) { // Correct approach: convert String to integer before comparing
// This code will execute.
}
3. Incorrect Logical Operator Usage
Misusing logical operators can lead to incorrect boolean evaluations. Double-check the logic to ensure it aligns with the desired behavior.
Example (Python):
x = 10
y = 5
z = 20
if x > y and x < z: # Correct logic. This will be true.
print("Condition is True")
if x > y or x > z: # Incorrect logic if you intended both conditions to be true. This will be true because x>y is true.
print("Condition is True, even though x is not greater than z")
if x > y and x > z: # Corrected logic. This will be false.
print("Condition is False")
4. Floating-Point Comparisons
Comparing floating-point numbers (like float
or double
in C++ or Java, or float
in Python) for equality is generally discouraged due to potential rounding errors. Instead, use a tolerance range to check for near-equality.
Example (C#):
float a = 0.1f + 0.2f; // Might not be exactly 0.3 due to floating-point representation
float b = 0.3f;
// Avoid this:
if (a == b) { // This might be false due to rounding errors
}
// Instead, use a tolerance:
const float tolerance = 0.0001f;
if (Math.Abs(a - b) < tolerance) { // Check if the difference is within the tolerance
// This is a more robust approach.
}
5. Empty or Null Values
Checking for empty strings, null values, or empty collections requires specific checks. Directly comparing these values to other values might lead to unexpected results or errors.
Example (PHP):
$name = "";
if ($name == "John") { // This will be false because an empty string is not equal to "John".
}
if (empty($name)) { // Correctly checks for an empty string.
// This code will execute.
}
Example (Javascript):
let user = null;
if (user === "Alice"){ // This will be false because null is not equal to "Alice".
// This code will not execute.
}
if (user === null){ // This will be true.
//This code will execute.
}
6. Short-Circuiting in Logical Operators
Logical operators (and
, or
) exhibit short-circuiting behavior. In an and
expression, if the first operand is false, the second operand is not evaluated. Similarly, in an or
expression, if the first operand is true, the second operand is not evaluated. Understanding this behavior is crucial for avoiding unintended side effects.
Example (Python):
def func1():
print("func1 called")
return False
def func2():
print("func2 called")
return True
if func1() and func2(): # func2 will not be called because func1() is False.
print("Both are True")
if func1() or func2(): # func2 will be called because func1() is False.
print("At least one is True")
Best Practices for Avoiding False Conditionals
To minimize the occurrence of false conditional statements, follow these best practices:
- Use the correct comparison operators: Carefully choose the appropriate operator (
==
,!=
,>
,<
,>=
,<=
) to reflect the desired comparison. - Ensure type consistency: Convert values to the same data type before comparing them to avoid type mismatch errors.
- Handle null or undefined values: Explicitly check for null or undefined values using appropriate methods before performing comparisons.
- Test thoroughly: Comprehensive testing is crucial to identify scenarios where conditional statements might unexpectedly evaluate to false.
- Use descriptive variable names: This improves code readability and reduces the chance of logical errors.
- Break down complex conditions: Decompose complex conditions into smaller, more manageable parts for better understanding and debugging.
- Use debugging tools: Utilize debuggers to step through code execution and inspect variable values to understand why a conditional statement might be returning false.
- Code reviews: Peer reviews can help identify potential issues in your logic.
Conclusion
Understanding when a conditional statement evaluates to false is paramount for developing reliable software. By carefully examining comparison operators, logical operators, data types, and edge cases, you can effectively write code that functions correctly under diverse circumstances. Consistent application of best practices and thorough testing are essential for preventing unexpected false conditionals and ensuring the robustness of your software. Mastering boolean logic and its implications is a crucial step towards becoming a proficient programmer. Remember to always double-check your logic, especially when dealing with complex conditions and edge cases.
Latest Posts
Related Post
Thank you for visiting our website which covers about When Is A Conditional Statement False . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.