How To Multiply Vector Wise Equations
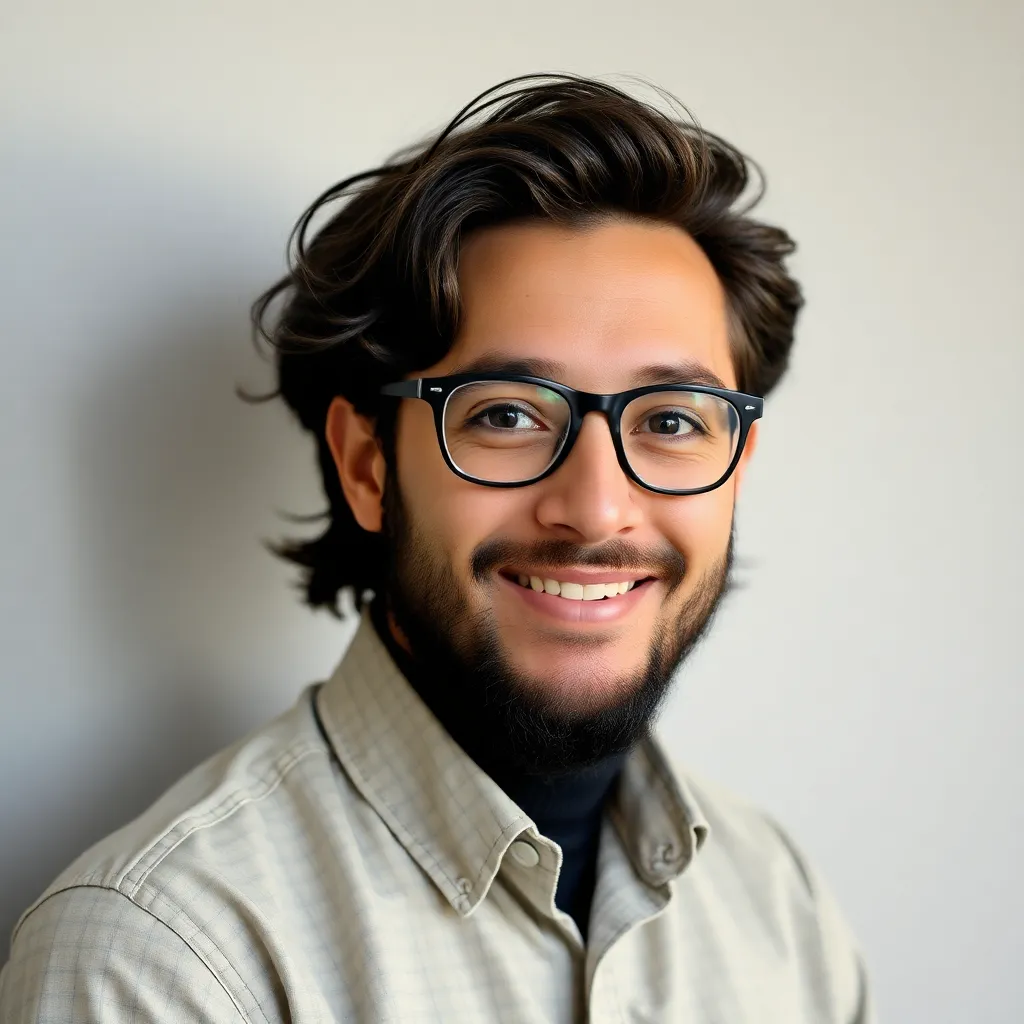
Muz Play
Mar 24, 2025 · 5 min read
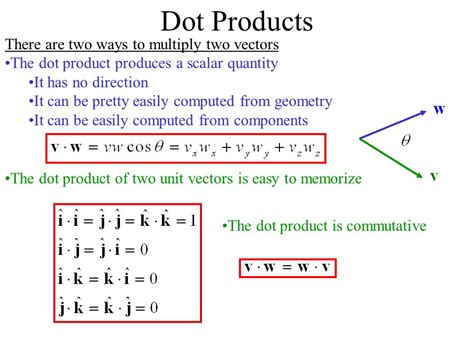
Table of Contents
How to Multiply Vector-Wise Equations: A Comprehensive Guide
Vectorization, the process of performing operations on entire arrays or vectors instead of individual elements, is a cornerstone of efficient numerical computation. It significantly speeds up calculations and simplifies code, especially when dealing with large datasets common in fields like machine learning, physics, and computer graphics. This article delves deep into the intricacies of multiplying vector-wise equations, covering various scenarios and techniques.
Understanding Vector-Wise Operations
Before tackling multiplication, it's crucial to grasp the fundamental concept of vector-wise operations. Unlike scalar operations that involve single numbers, vector-wise operations apply an operation to corresponding elements of vectors or matrices. Consider the following examples:
- Element-wise addition:
[1, 2, 3] + [4, 5, 6] = [5, 7, 9]
Each element in the first vector is added to its corresponding element in the second vector. - Element-wise multiplication (Hadamard product):
[1, 2, 3] * [4, 5, 6] = [4, 10, 18]
Elements are multiplied pairwise. - Dot product:
[1, 2, 3] ⋅ [4, 5, 6] = (1*4) + (2*5) + (3*6) = 32
This is a different operation resulting in a scalar value.
These examples highlight the distinction between element-wise operations and other vector operations. Understanding this distinction is paramount when dealing with vector-wise multiplication in equations.
Types of Vector-Wise Multiplication in Equations
Vector-wise multiplication within equations can manifest in several ways, depending on the context and the type of vectors involved. Let's explore the common scenarios:
1. Element-wise Multiplication in Linear Equations
Consider a simple linear equation: y = mx + c
, where 'm' and 'c' are scalars and 'x' and 'y' are vectors. To perform vector-wise multiplication, we apply the scalar multiplication to each element of the vector 'x' and then add the scalar 'c' to each element of the resulting vector.
Example:
Let m = 2
, c = 1
, and x = [1, 2, 3]
. Then:
y = 2 * [1, 2, 3] + 1 = [2, 4, 6] + 1 = [3, 5, 7]
This operation is efficiently handled using libraries like NumPy in Python or similar vectorized libraries in other programming languages.
2. Hadamard Product in Equations
When dealing with multiple vectors of the same dimension, the Hadamard product (element-wise multiplication) becomes relevant. For example, consider the equation: z = x * y
, where '*' denotes the Hadamard product.
Example:
Let x = [1, 2, 3]
and y = [4, 5, 6]
. Then:
z = [1, 2, 3] * [4, 5, 6] = [4, 10, 18]
This type of multiplication is frequently used in machine learning for tasks such as masking arrays or applying weights element-wise.
3. Matrix-Vector Multiplication
In more complex scenarios, we might encounter matrix-vector multiplication. This involves multiplying a matrix by a vector, resulting in a new vector. This operation isn't strictly element-wise but is a fundamental operation in linear algebra and is heavily used in vectorized computations.
Example:
Let's say we have a matrix A
and a vector x
:
A = [[1, 2],
[3, 4]]
x = [5, 6]
The matrix-vector product Ax
is calculated as:
Ax = [[(1*5) + (2*6)],
[(3*5) + (4*6)]] = [[17],
[39]]
This operation is efficiently implemented in linear algebra libraries.
4. Vector-Wise Multiplication with Functions
Vector-wise operations extend beyond basic arithmetic. We can apply arbitrary functions element-wise to vectors.
Example:
Let's say we want to calculate the square root of each element in a vector x = [1, 4, 9]
. We can express this as:
y = sqrt(x) = [1, 2, 3]
Most programming languages and numerical libraries provide efficient functions for applying arbitrary functions element-wise to vectors or matrices.
Implementing Vector-Wise Multiplication in Different Programming Languages
The implementation of vector-wise multiplication varies slightly across programming languages, but the core concepts remain consistent.
Python with NumPy
NumPy is the de facto standard for numerical computation in Python. It provides highly optimized functions for vectorized operations.
import numpy as np
x = np.array([1, 2, 3])
y = np.array([4, 5, 6])
# Element-wise multiplication
z = x * y # Output: array([ 4, 10, 18])
# Matrix-vector multiplication
A = np.array([[1, 2], [3, 4]])
result = np.dot(A, x) # Output: array([14, 39])
#Applying a function element-wise
sqrt_x = np.sqrt(x) # Output: array([1. , 1.41421356, 1.73205081])
MATLAB
MATLAB is another powerful environment for numerical computation that inherently supports vectorized operations. Its syntax is very similar to the element-wise operations shown above using NumPy.
Other Languages
Languages like R, Julia, and C++ (using libraries like Eigen) also offer efficient mechanisms for performing vector-wise operations. The core concepts of element-wise operations and matrix-vector multiplication are generally consistent across these languages.
Advanced Techniques and Considerations
Broadcasting
Broadcasting is a powerful feature in many numerical libraries (like NumPy) that automatically expands the dimensions of arrays to make element-wise operations possible even when arrays have different shapes. This simplifies code and avoids explicit looping.
Performance Optimization
For extremely large datasets, performance optimization is critical. Techniques such as using optimized linear algebra libraries (like BLAS and LAPACK) and parallelization can significantly improve the speed of vector-wise computations.
Memory Management
When dealing with massive vectors or matrices, careful memory management is crucial to avoid memory exhaustion. Techniques such as memory mapping or using specialized data structures can be necessary.
Conclusion
Vector-wise multiplication is a fundamental concept in numerical computation, enabling efficient and concise code for various applications. Understanding the different types of vector-wise multiplication, choosing the appropriate technique based on the problem at hand, and utilizing the optimized libraries available in various programming languages are key to writing efficient and scalable code. Mastering these techniques is essential for anyone working with large datasets or in fields heavily reliant on numerical computation. Through careful consideration of data structures, algorithmic efficiency, and available libraries, you can leverage the power of vectorization to significantly improve the performance and readability of your code. Remember to always choose the most appropriate method depending on the context of your equation and the desired outcome. Experiment with different approaches and libraries to discover which methods best suit your specific needs and performance requirements.
Latest Posts
Latest Posts
-
How To Multiply And Divide Rational Expressions
Mar 29, 2025
-
Difference Between Lewis Acid And Bronsted Acid
Mar 29, 2025
-
Which Of The Following Orbitals Is The Last To Fill
Mar 29, 2025
-
What Is A Fluid Connective Tissue
Mar 29, 2025
-
What Is The Van Der Waals Equation
Mar 29, 2025
Related Post
Thank you for visiting our website which covers about How To Multiply Vector Wise Equations . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.